Invoke An Azure Runbook Utilizing Terraform
Introduction
Let’s soar into the issue that we now have – we wish to invoke certainly one of our Azure Automation Runbooks from Terraform to carry out a selected process the place we needed to move some parameters that had been required for runbook execution. We’re going to state the apparent and it could come as a shock to a few of you – like another instruments/platforms, Terraform has its personal set of limitations and is understood for provisioning new sources solely, It doesn’t provide a direct option to invoke runbooks by passing required parameters.
With the choices out there in Terraform for runbooks, you possibly can solely create a brand new runbook with new schedule however can’t carry out operations like invoking an present runbook by passing required parameter values, utilizing terraform sources. So, we needed to search for different choices out there in Terraform. Guess what we received….local-exec provisioner. With this local-exec provisioner, we tried to meet our necessities. Allow us to clarify how we carried out this in order that others can reuse this prepared to make use of stuff and might keep away from spending hours on the identical factor. The reusable code for this use case is on the market right here: Invoke-Azure-Runbooks.
Pre-Requisites
- Azure Automation Account
- PowerShell Runbook hosted on the above automation account
- Terraform Open Supply or Enterprise Model
Runbook Implementation
For the sake of simplicity, we will likely be explaining a easy state of affairs the place you possibly can invoke a runbook by passing the required parameters to it from Terraform and runbook will output the values of handed parameters. As talked about above, there are various kinds of Runbooks however for this weblog, we will likely be specializing in PowerShell sort runbook. In case you are new to PowerShell, please take a look at this superb article for extra particulars on Microsoft PowerShell. We’re not detailing the runbook implementation as it could differ primarily based on use circumstances or necessities and the aim of this text is to elucidate runbook invocation by way of terraform by passing parameters solely.
Let’s start with implementation. The primary order of motion is to create a runbook within the Azure Automation account and a easy PowerShell script for it which accepts parameters.
Please observe the beneath steps:
- Open your Automation account in Azure Portal.
- Click on Runbooks underneath Course of Automation. The listing of runbooks is displayed.
- Click on Create a runbook on the prime of the listing.
- Enter a reputation for the runbook identify within the Identify area and choose PowerShell for the Runbook sort area.
- Click on on the Create button to create a runbook.
-
Now, on “Edit PowerShell Runbook”, copy and paste beneath PowerShell code.
- param(
- [Parameter(Mandatory = $true)]
- [object] $WebhookData)
- attempt {
- # If runbook was referred to as from Webhook, WebhookData will not be null.
- if($WebhookData) {
- #Retrieve knowledge from Webhook request physique
- $inputs = (ConvertFrom – Json – InputObject $WebhookData.RequestBody)
- #Fetch values of parameters handed to webhook in kind of request physique
- $Attribute1 = $inputs.Attribute1
- $Attribute2 = $inputs.Attribute2
- Write – Output “==========================================================”
- Write – Output “Worth of First Parameter – “
- $Attribute1
- Write – Output “Worth of Second Parameter – “
- $Attribute2
- Write – Output “==========================================================”
- }
- } catch {
- Write – Output $_.Exception.Message
- Write – Error “This runbook is meant to be began from webhook solely.” – ErrorAction Cease
- }
- Click on on Save after which publish buttons seen on the identical display screen.
- Now, click on on Webhook button out there on the revealed runbook display screen
- On the Add Webhook display screen, click on on Create a brand new Webhook possibility.
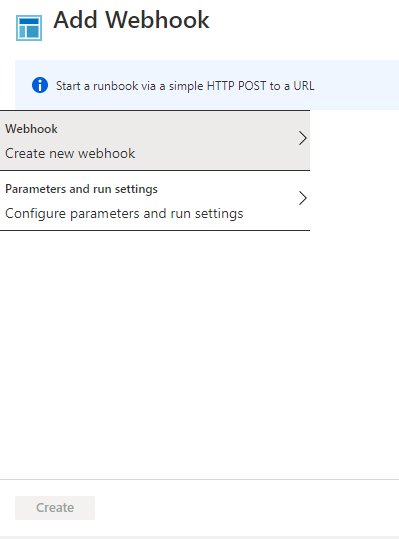
- On the subsequent display screen, present a reputation within the Identify area and expiry DateTime to your Webhook. Copy the Webhook Url and maintain it apart as it will likely be utilizing in Terraform for invoking the runbook
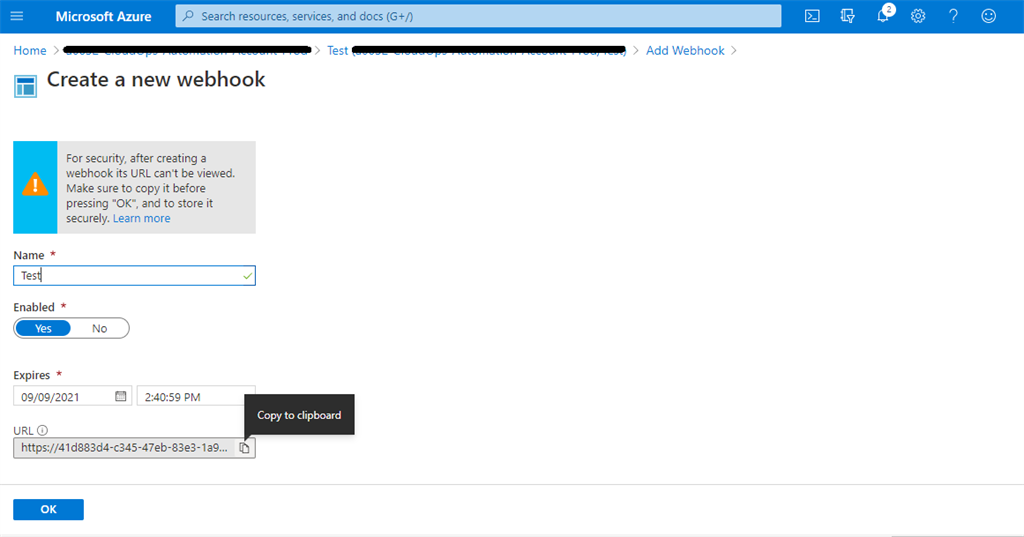
- Now, Click on on OK after which Create.
Now we’re all set with our runbook that may be invoked from anyplace utilizing its Webhook URL.
Terraform Implementation
We are going to observe the beneath code hierarchy that we adopted in our earlier article the place every deployment sort has a separate folder containing all required recordsdata together with a principal file, a variables file, an output file, and an elective .tfvars file that gives inputs for executions.
principal.tf
Let’s start with Terraform implementation by opening the primary.tf file and including the next code block. As we talked about within the above sections, Terraform doesn’t help the invocation of runbooks by means of its native sources and due to this fact, we will likely be utilizing a “local-exec” provisioner with a “null_resource” useful resource the place a PowerShell script will likely be used to invoke our runbook created within the above steps.
- #Invocation of Azure Runbook by way of Native – Exec
- useful resource “null_resource”
- “invoke_azure_runbook” {
- provisioner “local-exec” {
- command = “.Invocation-Script.ps1 -Param1 ‘${var.variable1}’ -Param2 ‘${native.variable2}'”
- interpreter = [“pwsh”, “-Command”]
- }
- }
Right here, we’re making a “null_resource” useful resource of Terraform with “local-exec” provisioner used for executing a PowerShell script i.e. “Invocation-Script.ps1” by passing the arguments to it from variable.tf file or native variables. Now, we will likely be including a locals block to outline the native variables that we utilized in above code. On this block, we’re manipulating the “resource_group_name” variable worth a bit, handed from variable.tf file and passing the manipulated worth as a neighborhood variable worth to the local-exec provisioner.
We’re including this block to indicate that we are able to additionally move native variables (manipulated variables) accountable for the “local-exec” provisioner as argument values.
- #Outline Native Variables
- locals {
- #Changing clean areas from variable worth and storing it in native variable referred to as“ variable2”
- variable2 = exchange(var.variable2, ” “, “”)
- }
Now, let’s create “Invocation-Script.ps1” PowerShell file, add it to the basis of your Terraform listing the place all different recordsdata like principal.tf, variable.tf are positioned and add the next easy code.
- # Outline Parameters to be handed to script from Terraform by way of Native – Exec
- param(
- [string] $Param1,
- [string] $Param2)
- #Create a request physique object
- for webhook
- $physique = @ {
- Attribute1 = $Param1
- Attribute2 = $Param2
- }
- #Convert above object into Json
- $requestBody = $physique | ConvertTo – Json
- $uri = “Your_Runbook_Webhook_Uri which you copied throughout webhook creation”
- #Invoke the webhook utilizing Webhook Uri
- $response = Invoke – WebRequest – Methodology Submit – Uri $uri – Physique $requestBody
- if ($response.StatusCode – eq “202” – and $response.StatusDescription – eq “Accepted”) {
- Write – Host “Your webhook invocation is accomplished.”
- } else {
- Write – Host “Your webhook invocation has failed.”
- }
This “Invocation-Script.ps1” is accepting the parameter values that are handed from Terraform to it as argument values and is invoking the webhook utilizing the “Invoke-WebRequest” command of PowerShell and passing the parameter values as Webhook request physique in Json format.
The principle executable code is now prepared and we are going to simply create the 2 further recordsdata for variables and output to outline the enter and output variables that will likely be a part of this execution.
variables.tf
- variable variable1 {
- sort = string
- description = “First variable to be handed to Terraform”
- default = “Runbook Invocation”
- }
- variable variable2 {
- sort = string
- description = “First variable to be handed to Terraform”
- default = “By way of Terraform”
- }
outputs.tf
As “null_resource” doesn’t return output of executed PowerShell script, so we are able to have a customized output saved in a variable that will likely be depending on “null_resource” useful resource execution completion.
- output “Remarks” {
- worth = “Your runbook has been invoked efficiently”
- depends_on = [
- null_resource.invoke_azure_runbook
- ]
- }
That’s it!! We’re prepared with the code now that may invoke runbook by means of webhook by passing required parameters from Terraform. As talked about in our earlier articles additionally, bear in mind to carry out a Terraform Init earlier than doing a plan and apply. We’re utilizing a pattern tfvars file for our native execution with variables outlined underneath,
- variable1 = “your-value-for-variable1”
- variable2 = “your-value-for-variable2”
- terraform plan –var-file=“<yourfilename>.tfvars” or terraform plan
- terraform apply –var-file=“<yourfilename>.tfvars” or terraform apply
As soon as you’ll run the “Terraform Apply” operation, it offers you beneath output:
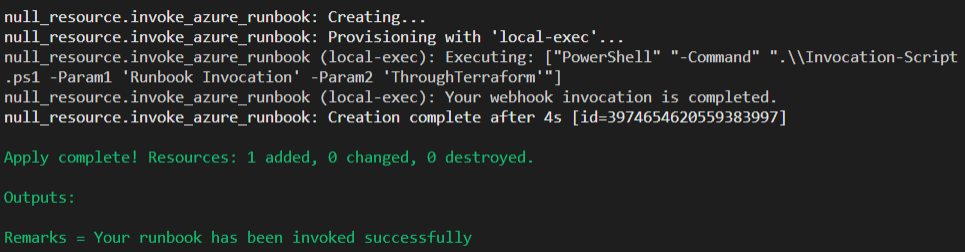
If you’ll go to the runbook jobs at Azure Portal, following output will likely be displayed in underneath “All Logs” in opposition to job executed out of your webhook invocation.
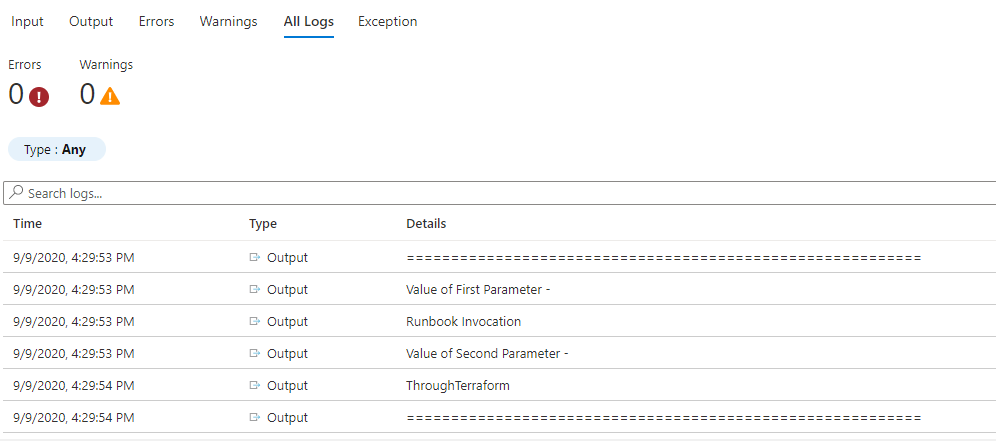